For the last 2 days, I thought of unleashing few of the hidden facts of Data Storage. It is true, that introduction of .NET framework without introducing Generic in first place is one of the biggest mistakes. Because of Non-Generic data structure a large amount of data is been unnecessarily boxed and unboxed without any reason as such. After Generics was introduced with .NET 2.0, most of these classes which made you do type conversions is either been totally depreciated or few of them rarely used as is there for backward compatibility. But for every programmer, the most common class called DataTable still exists without any change. In this post we will try to see the actual implementation of DataTable as a whole, so that we could understand what is going on when we store data into it.
Introduction
DataTable is an application object that maps to a Database Table. It holds a collection of DataRows and DataColumns where DataRow represents the actual Data while the DataColumns holds Integration rules. Basically we all know the basics of DataTable and often use it for our regular work.
DataSet holds a list of DataTable, and DataRelation. DataTable as being said is the storage of Data in form of a Table with Row and Columns, the DataRelation holds the Relationship rules between two tables in a DataSet. Hence ADO.NET has been structured in such a way that you can have the flavour of entire database from your application end.
But, one thing that you must keep in mind, these objects are created way back in time of .NET 1.0 release or even before when there is no Generics or even the concept of State Machines. Generics allows you to pass type of an object during actual object creation and hence will allow you to create Type information based on the object which we create in real time, as a result it eliminates unnecessary runtime boxing and unboxing. Keeping this in mind, I thought I should check how these classes are made in Framework. Lets look each of them, one by one in this post and lets identify pros and cons.
Read Disclaimer Notice
ADO.NET : Some internals uncovered Part 2
Posted by
Abhishek Sur
on Friday, December 31, 2010
Labels:
.NET,
ADO.NET,
C#,
CodeProject
0
comments
WPF Tutorial
Posted by
Abhishek Sur
on Wednesday, December 29, 2010
Labels:
.NET,
C#,
WPF
0
comments
WPF, a.k.a Windows Presentation Foundation provides an unified model for producing high end graphical business application easily using normal XML syntax (known as XAML) which runs both in client and server leveraging the job to render output more relying on graphics devices rather than using GDI components.
If you are looking to learn WPF, its time to start on today. I have put all my articles on WPF in Codeproject, which you might find interesting and helpful.
Article Links :
If you are looking to learn WPF, its time to start on today. I have put all my articles on WPF in Codeproject, which you might find interesting and helpful.
Article Links :
- WPF Tutorial : Beginning [^]
- WPF Tutorial : Layout-Panels-Containers & Layout Transformation [^]
- WPF Tutorial : Fun with Border & Brush [^]
- WPF Tutorial - TypeConverter & Markup Extension [^]
- WPF Tutorial - Dependency Property [^]
- WPF Tutorial - Concept Binding [^]
- WPF Tutorial - Styles, Triggers & Animation [^]
I hope you would like those articles, comment on them and give feedback to them.
Thank you.
Read Disclaimer Notice
Thank you.
Internals of a Delegate
Posted by
Abhishek Sur
on Sunday, December 26, 2010
Labels:
.NET,
C#,
CodeProject,
Database,
internals
3
comments
Strange Rules of Delegate
Well, at recent times, at least after the introduction of .NET framework 3.5 the use of Delegates in a program has increased quite a bit. Now almost every people in .NET language must at least somehow used delegates on their daily programming activities. It might be because of the fact that the use of delegates has been simplified so much with the introduction of lambda expressions in .NET framework 3.5 and also the flexibility to pass delegates over other libraries for decoupling and inversion of control in applications. Hence, we can say the way of writing code in .NET environment has been changed considerably in recent times.
Introduction
If you want the most simple and somewhat vague idea about delegates I would say a delegate is actually a reference to a method so that you might use the reference as you use your object reference in your code, you can send the method anywhere in your library or even pass to another assembly for its execution, so that when the delegate is called, the appropriate method body will get executed. Now, to know a more concrete and real life example, I must consider you to show a code :
In the above code, I have explicitly declared a delegate and named it mydelegate. The name of the delegate will indicate that it is a type that can create reference to a method which can point to a method which have same signature as defined in it. Clearly If you quickly go through the code defined above, the property YourMethod can point to a signature which has same signature as declared to mydelegate. Hence, I can pass a method CallMe easily to Type A, so that when the object of A calls the delegate, it executes the method CallMe.
Read Disclaimer Notice
Well, at recent times, at least after the introduction of .NET framework 3.5 the use of Delegates in a program has increased quite a bit. Now almost every people in .NET language must at least somehow used delegates on their daily programming activities. It might be because of the fact that the use of delegates has been simplified so much with the introduction of lambda expressions in .NET framework 3.5 and also the flexibility to pass delegates over other libraries for decoupling and inversion of control in applications. Hence, we can say the way of writing code in .NET environment has been changed considerably in recent times.
Introduction
If you want the most simple and somewhat vague idea about delegates I would say a delegate is actually a reference to a method so that you might use the reference as you use your object reference in your code, you can send the method anywhere in your library or even pass to another assembly for its execution, so that when the delegate is called, the appropriate method body will get executed. Now, to know a more concrete and real life example, I must consider you to show a code :
public delegate int mydelegate(int x); public class A { public mydelegate YourMethod { get; set; } public void ExecuteMe(int param) { Console.WriteLine("Starting execution of Method"); if (this.YourMethod != null) { Console.WriteLine("Result from method : {0}", this.YourMethod(param)); } Console.WriteLine("End of execution of Method"); } } static void Main(string[] args) { //int x = 20; //int y = 50; A a1 = new A(); a1.YourMethod = Program.CallMe; a1.ExecuteMe(20); Console.Read(); } public static int CallMe(int x) { return x += 30; } }
In the above code, I have explicitly declared a delegate and named it mydelegate. The name of the delegate will indicate that it is a type that can create reference to a method which can point to a method which have same signature as defined in it. Clearly If you quickly go through the code defined above, the property YourMethod can point to a signature which has same signature as declared to mydelegate. Hence, I can pass a method CallMe easily to Type A, so that when the object of A calls the delegate, it executes the method CallMe.
Read Disclaimer Notice
ADO.NET - Some Internals Uncovered
Posted by
Abhishek Sur
on Sunday, December 19, 2010
Labels:
.NET,
ADO.NET,
CodeProject,
Database,
internals
0
comments
During the early phaze of your career, when you just have started learning about .NET technology, you might have came across the sentence "ADO.NET is a disconnected database architecture". Well, its true, but how? How can ADO is transformed to such an architecture which no other database architecture supports. Every other database programming supports only
In this post, I will uncover few internals of ADO.NET architecture, and also refer to my favorite tool Reflector to see the code that is written inside the .NET classes. My focus in the post is only on the internals of the architecture, so if you are new to ADO.NET, it would not be a good idea to read it over and confuse yourself more, rather read thorough Overview of ADO.NET and come back later.
Introduction
Well, In fact, I didn't ever thought of writing such a post. Strangely, while talking with Devs I found out people commenting that ADO.NET is a new way of accessing data missing out the point where ADO.NET differs with ADO. It is true, ADO.NET is disconnected. There are large performance gain of an application which releases the Connection after fetching data from the database. ADO.NET have inherent capability to release the database connection, after the so called DataSet / DataTable is filled with data which you might be looking for. There are few Adapters provided with .NET library which lets you Fill these DataSet or DataTable when you want and later you can use these cached data to your application. Yes every line here is a fact. But internally there is still a Reader associated with every call. Let me detail the fact a little.
Details of Loading Data using Select Query
Say you invoke a Selection operation on the Database using Select Query. Lets jot down the steps what happens internally :
Read Disclaimer Notice
RecorsSet
which you need to use to get Data from the database. Did you ever thought of it ? If you don't, it is time to rethink of it now.In this post, I will uncover few internals of ADO.NET architecture, and also refer to my favorite tool Reflector to see the code that is written inside the .NET classes. My focus in the post is only on the internals of the architecture, so if you are new to ADO.NET, it would not be a good idea to read it over and confuse yourself more, rather read thorough Overview of ADO.NET and come back later.
Introduction
Well, In fact, I didn't ever thought of writing such a post. Strangely, while talking with Devs I found out people commenting that ADO.NET is a new way of accessing data missing out the point where ADO.NET differs with ADO. It is true, ADO.NET is disconnected. There are large performance gain of an application which releases the Connection after fetching data from the database. ADO.NET have inherent capability to release the database connection, after the so called DataSet / DataTable is filled with data which you might be looking for. There are few Adapters provided with .NET library which lets you Fill these DataSet or DataTable when you want and later you can use these cached data to your application. Yes every line here is a fact. But internally there is still a Reader associated with every call. Let me detail the fact a little.
Details of Loading Data using Select Query
Say you invoke a Selection operation on the Database using Select Query. Lets jot down the steps what happens internally :
Read Disclaimer Notice
Ways of Throwing an Exception in C#
Posted by
Abhishek Sur
on Tuesday, December 7, 2010
Labels:
.NET,
.NET 3.5,
.NET 4.0,
.NET Memory Management,
C#,
CodeProject
4
comments
Exception handling is very common for everyone. Exceptions are runtime errors which might be caused by some operation illegal to the application. .NET provides a good Exception Model (even though Microsoft wants to change this model) using try/catch which lets us to deal with runtime exceptions from our code.
It is stated that, you write your code that might generate an exception inside try block, write the logic which handles the exception generated within the catch block ( like logging, notifying etc.), where catch block can overload. Also you should keep in mind, it is recommended to wrap your code in try / catch only if you think you can handle the exception, if you cannot, it is better to leave apart the exception and let the caller to handle this in their own code. Lets take an example :
So in the above code the Try/catch block is introduced to implement the code with exception handling. I am using DumpException which writes the exception to the Console.
Download Sample Code - 30 KB
Read Disclaimer Notice
It is stated that, you write your code that might generate an exception inside try block, write the logic which handles the exception generated within the catch block ( like logging, notifying etc.), where catch block can overload. Also you should keep in mind, it is recommended to wrap your code in try / catch only if you think you can handle the exception, if you cannot, it is better to leave apart the exception and let the caller to handle this in their own code. Lets take an example :
public void FirstCall() { try { this.SecondCall(); Console.WriteLine("After Exception"); } catch (Exception ex) { this.DumpException(ex); } }
So in the above code the Try/catch block is introduced to implement the code with exception handling. I am using DumpException which writes the exception to the Console.
Download Sample Code - 30 KB
Read Disclaimer Notice
Win32 Handle (HWND) & WPF Objects - A Note
Posted by
Abhishek Sur
on Sunday, December 5, 2010
Labels:
.NET 4.0,
C#,
CodeProject,
WPF
0
comments
Well, there is a common confusion for many who are working with WPF objects, is does it truly interoperable with Windows environment, or more precisely, does it act the same way as it does with normal Win32 objects?
The problem here comes with the fact that each WPF window has only one window handle (HWND) and each other controls are actually placed as content to the window and does not have any entry on Kernel table(no HWND) except of course Popup class in WPF. In this post I will try to cover the basis of HWND (for those of you who don't know) and later go on with what are the changes of it with WPF environment.
If you know what HWND is or how it works, please skip the next section.
Overview of Win32 HANDLE
Win32 Handle is very important for any Win32 applications. For every Win32 apps, Kernel maintains a Table which has entries to identify a memory address. A HANDLE is actually a DWORD (32 bit integer) which maps the memory address on the table. So if you get a HANDLE, you can easily locate the memory address it points to. Each object that you have in Windows (like windows, buttons, mouse pointers, icon, menu, bitmap etc) has entries in the table and the object is traced using both internally by windows or by programs using those HANDLEs. Even though it is just an unsigned integer value, you should not edit the value, otherwise the HANDLE could not be used to point the object anymore.
Read Disclaimer Notice
The problem here comes with the fact that each WPF window has only one window handle (HWND) and each other controls are actually placed as content to the window and does not have any entry on Kernel table(no HWND) except of course Popup class in WPF. In this post I will try to cover the basis of HWND (for those of you who don't know) and later go on with what are the changes of it with WPF environment.
If you know what HWND is or how it works, please skip the next section.
Overview of Win32 HANDLE
Win32 Handle is very important for any Win32 applications. For every Win32 apps, Kernel maintains a Table which has entries to identify a memory address. A HANDLE is actually a DWORD (32 bit integer) which maps the memory address on the table. So if you get a HANDLE, you can easily locate the memory address it points to. Each object that you have in Windows (like windows, buttons, mouse pointers, icon, menu, bitmap etc) has entries in the table and the object is traced using both internally by windows or by programs using those HANDLEs. Even though it is just an unsigned integer value, you should not edit the value, otherwise the HANDLE could not be used to point the object anymore.
Read Disclaimer Notice
Summary of Microsoft Community Tech Days - 28th November Kolkata
Posted by
Abhishek Sur
on Monday, November 29, 2010
Labels:
ASP.NET 4.0,
async,
C#,
debugging,
Online Session,
WPF
2
comments
Yesterday(28th November 2010), we had our Session for Community Tech Days Kolkata, In association with Kolkata .NET community we have put our effort to produce few great Sessions from few great people. In this post, lets cherish those magical moments we had together (or if you missed). Here is the Agenda :
Read Disclaimer Notice
Read Disclaimer Notice
Debugging with Async
Posted by
Abhishek Sur
on Saturday, November 20, 2010
Labels:
async,
C#,
C#5.0
0
comments
C# async feature really exposes a lots of things to us. I can see, there is lots of people is discussing about it in MSDN forums, few people wanted to get rid of the Task from the async methods and really want to deal with normal return types, while others just wanted to get rid of the async postfix. During my leisure, I read them but what I thought the most important part of the feedback is that most of the people really liked the way Microsoft simplifies the Asynchronous approach of programming style.
I have already studied some of the important facts on Async style of programming, and found that it uses the StateMachine to let the program return after a certain portion of the method gets executed while the Rest of the program is sent as ContinueWith for the Task. Thus the TaskCompletion automatically restarts the method and continue. If you want to know more about how Task gets executed in async methods, please read my article where I have discussed how exactly the current trend of programming style got changed.
Today while I was looking at the implementation of Async style of programming, I just discovered an Internal class named DebugInfo inside the System.Runtime.CompilerServices.AsyncMethodBuilder.
You can see the class is internal, and hence not exposed from the perspective of BCL. You cannot create object of the class or even look at the private member LocationForDebuggerDisplay. But the only thing that you can see through this is the ActiveMethods.
Read Disclaimer Notice
I have already studied some of the important facts on Async style of programming, and found that it uses the StateMachine to let the program return after a certain portion of the method gets executed while the Rest of the program is sent as ContinueWith for the Task. Thus the TaskCompletion automatically restarts the method and continue. If you want to know more about how Task gets executed in async methods, please read my article where I have discussed how exactly the current trend of programming style got changed.
Today while I was looking at the implementation of Async style of programming, I just discovered an Internal class named DebugInfo inside the System.Runtime.CompilerServices.AsyncMethodBuilder.
You can see the class is internal, and hence not exposed from the perspective of BCL. You cannot create object of the class or even look at the private member LocationForDebuggerDisplay. But the only thing that you can see through this is the ActiveMethods.
Read Disclaimer Notice
Microsoft Community Tech Days - 28th November
Posted by
Abhishek Sur
on Sunday, November 14, 2010
Labels:
Online Session,
WPF
1 comments
Microsoft is going to arrange a Community Technical Day for us on 28th November 2010 here in Kolkata. The whole thing is organized by Kolkata Dotnet Community. I am very glad to announce that I am going to present Ribbon UI control for the seminar.
I will mainly present Windows Presentation Foundation in light of a new Ribbon UI Controlset introduced recently and may be added to the main line library in next version of WPF. Be there, if you wish.
To Register :
Who else is speaking?
Abu Sayed Mohammad Ismail, Sankarsan Bose, Rajiv Popat, Abhijit Jana
See you there. Read Disclaimer Notice
I will mainly present Windows Presentation Foundation in light of a new Ribbon UI Controlset introduced recently and may be added to the main line library in next version of WPF. Be there, if you wish.
To Register :
![]() |
Register Yourself |
Who else is speaking?
Abu Sayed Mohammad Ismail, Sankarsan Bose, Rajiv Popat, Abhijit Jana
See you there. Read Disclaimer Notice
C# 5.0 vNext - Asynchrony in Synchronous flow style code
Posted by
Abhishek Sur
Labels:
C#5.0,
PDC10
0
comments
There is a buzz all around on the new way of doing asynchronous programming using async database modifier and await contextual keyword. Threading or Asynchronous style of programming is very useful at times but each of us faced a lots of problems though converting a Synchronous code to Asynchronous coding style. Asynchronous style of coding is not new to the framework. From the very beginning of the evolution of C# as a language we can do programs that may run in parallel. But was it simple enough? No ! Its not. The way of writing our very own asynchronous programs can be very complex at times when we are about to deal with exception handling. We could have lots of callbacks around for every single asynchronous calls, there might be few thread blocks around for network calls etc.
C# 5.0 takes this into consideration and orchestrate the style of coding to more like synchronous manner.
I have already introduced, the two new keywords async and await which was introduced with async CTP recently, but it was just a rough introduction to the fact (as I posted that on the same day). Now let me move a bit further in this world and go with more complete asynchronous style of coding.
I have put a lovely article for you in codeproject with few sample codes. Please try the link below to read the whole story around async and await :
C# 5.0 vNext - New Asynchronous Pattern
You can also download sample Code from
Sample-CodeAsync - 385KB
I hope you would like this article. Please give your comments and feedbacks.
Thank you. Read Disclaimer Notice
C# 5.0 takes this into consideration and orchestrate the style of coding to more like synchronous manner.
I have already introduced, the two new keywords async and await which was introduced with async CTP recently, but it was just a rough introduction to the fact (as I posted that on the same day). Now let me move a bit further in this world and go with more complete asynchronous style of coding.
I have put a lovely article for you in codeproject with few sample codes. Please try the link below to read the whole story around async and await :
C# 5.0 vNext - New Asynchronous Pattern
You can also download sample Code from
Sample-CodeAsync - 385KB
I hope you would like this article. Please give your comments and feedbacks.
Thank you. Read Disclaimer Notice
Reflection - Slow or Fast? Demonstration with Solutions
Posted by
Abhishek Sur
on Saturday, November 6, 2010
Labels:
.NET,
.NET 3.5,
.NET 4.0,
.NET Memory Management,
C#,
Reflection
5
comments
Many of you might have heard, calling reflection APIs from your code is always slower than that of calling it directly. Well, it is right. Some of the major Reflection methods like GetXXX (of MethodInfo, PropertyInfo, FieldInfo etc) are say 100 times slower than that of calling a Method, Property or Fields directly. In this post, I will try to cover some of the major portion of Reflection which is slower like hell than that of normal method call and also try to solve the same.
So what is Reflection for?
As I have already told you about Reflection and Code Emit and also how to build your Lamda Expression Tree, it is good to know where you would like to use Reflection. Yes... Reflection is made truly late bound approach to browse through the object hierarchy or to call methods inside the object. So if you do not know much about your object hierarchy or you have somehow found an assembly external to your application and you need to parse through the object and call its methods say, then Reflection would be the right choice for you.
So basically Reflection allows you to create plugin type of applications where you define an Interface and publish the same for your application and let others create those plugins for you to seamlessly added to your application. Fine. Then what it isn't for?
Yes basically few people try to work everything based on Reflection. Using reflection unnecessarily will let your application very costly. Say for instance,
Or say using
Silly huh..
I saw few people to do this. They try to use everything using Reflection, may be they love the Reflection too much, or they have already created a Reflection engine right and do not want to mix up unknown types with known ones. May be they would make their code clear by calling the known types to the same module that is built up for dealing with unknown types.
Download Sample - 60 KB
Read Disclaimer Notice
So what is Reflection for?
As I have already told you about Reflection and Code Emit and also how to build your Lamda Expression Tree, it is good to know where you would like to use Reflection. Yes... Reflection is made truly late bound approach to browse through the object hierarchy or to call methods inside the object. So if you do not know much about your object hierarchy or you have somehow found an assembly external to your application and you need to parse through the object and call its methods say, then Reflection would be the right choice for you.
So basically Reflection allows you to create plugin type of applications where you define an Interface and publish the same for your application and let others create those plugins for you to seamlessly added to your application. Fine. Then what it isn't for?
Yes basically few people try to work everything based on Reflection. Using reflection unnecessarily will let your application very costly. Say for instance,
ConstructorInfo cinfo= typeof(MyType).GetConstructor(new Type[] { typeof(int), typeof(string) }); object createdObject = cinfo.Invoke(new object[] { 10, "Abhishek" }); MyType xyz = createdObject as MyType;
Or say using
MyType typ = Activator.CreateInstance<MyType>();
Silly huh..
I saw few people to do this. They try to use everything using Reflection, may be they love the Reflection too much, or they have already created a Reflection engine right and do not want to mix up unknown types with known ones. May be they would make their code clear by calling the known types to the same module that is built up for dealing with unknown types.
Download Sample - 60 KB
Read Disclaimer Notice
Rate your calls: Type Constraint Or Type Evaluation
Posted by
Abhishek Sur
on Thursday, November 4, 2010
Labels:
.NET,
C#
0
comments
You must be thinking why I am coming with such a strange Tag line today. Actually if you go on reading you would definitely understand what I am speaking on. In my last post, I have pointed out that Tuple is implemented in a strange way. If you see the 8th implementation of Tuple class, the one with 8 generic parameters, you will see, it gives a restriction to the last parameter TRest to allow only Tuple.
But the strange thing is, the restriction is not open. The Tuple class does not have this restriction specified in its generic type parameter TRest. So you can even declare the last argument as string, but when your application actually tries to create an object of it, the application will throw an ArgumentException.
This seemed to be very weird to me. I was thinking why did Microsoft did not put this in Type argument, making ITuple (an internal implementation) public, or create a special sealed class, and expose the same as a constraint to Type argument. I think this could be answered only by BCL Team.
Download Sample Code - 30KB
Read Disclaimer Notice
But the strange thing is, the restriction is not open. The Tuple class does not have this restriction specified in its generic type parameter TRest. So you can even declare the last argument as string, but when your application actually tries to create an object of it, the application will throw an ArgumentException.
This seemed to be very weird to me. I was thinking why did Microsoft did not put this in Type argument, making ITuple (an internal implementation) public, or create a special sealed class, and expose the same as a constraint to Type argument. I think this could be answered only by BCL Team.
Download Sample Code - 30KB
Read Disclaimer Notice
Working with Tuple in C# 4.0
Posted by
Abhishek Sur
on Wednesday, November 3, 2010
Labels:
.NET,
.NET 4.0,
C#,
CodeProject
5
comments
After writing about C# 5.0, you might think why I moved a step behind with C# 4.0. Hey, I will definitely keep my promise to write a good blog on new async and await feature, but because of time it is taking a bit time to go on. C# 4.0 being officially released contains lots of thoughts and approaches that comes handy in many places. There are lots of new features introduced with C# 4.0 where a few of them are really very handy.
Today, I will write about a very handy object introduced in FCL named Tuple which can store n - number of values in it. Yes, you specify the type of each of those variables as generic parameters, and the object will create those values for you. Lets see how it works.
Using a Tuple
Base class library exposes two objects. It exposes the static class Tuple which allows you to get a number of Tuple instances based on the Static method Create, and a number of Tuple classes, each of which takes some specific number of Generic arguments.
Read Disclaimer Notice
Today, I will write about a very handy object introduced in FCL named Tuple which can store n - number of values in it. Yes, you specify the type of each of those variables as generic parameters, and the object will create those values for you. Lets see how it works.
Using a Tuple
Base class library exposes two objects. It exposes the static class Tuple which allows you to get a number of Tuple instances based on the Static method Create, and a number of Tuple classes, each of which takes some specific number of Generic arguments.
- Tuple.Create<t1>
- Tuple.Create<t1,t2>
- Tuple.Create<t1,t2,t3>
- Tuple.Create<t1,t2,t3,t4>
- Tuple.Create<t1,t2,t3,t4,t5>
- Tuple.Create<t1,t2,t3,t4,t5,t6>
- Tuple.Create<t1,t2,t3,t4,t5,t6,t7>
- Tuple.Create<t1,t2,t3,t4,t5,t6,t7,t8>
Read Disclaimer Notice
C# 5.0 Asynchronous Made Easy
Posted by
Abhishek Sur
on Friday, October 29, 2010
Labels:
.NET,
C#,
C#5.0,
PDC10
13
comments
After I introduced about Future Of C# 5.0 I was not aware of what Microsoft is thinking about it. But today after I saw PDC 2010 the main concern that Microsoft is pointing to on the release of C# 5.0 is the pain related to deal with asynchronous operations using Threads. It simplifies the existing MultiThreading model with Task based model, which now simplified with greater extent with the use of two new keywords yet not introduced as "await" and "async".
The History
Before the introduction of these features, we generally rely on traditional approaches of creating Thread from a ThreadPool and consuming a method which needed to be asynchronously called for. Again when the Thread finishes, or during the lifetime of the Thread we need callbacks to notify UI after a while, so that the user gets an impression that the background is updating the system. This breaks the way we write or the way we think of programming. The programmer needs to create the program to device in such a way that it handles continuity of the application by writing custom callbacks to the system or even use Dispatcher to update the UI thread from background. Thus the code becomes more and more complex as we add up more functionalities to the system.
The Present
In this PDC, Anders Hejlsberg introduces two new investments on C# which is probably now still in CTP to allow asynchronous calls to the applications very simple and easy to handle. The two keyword which were introduced recently are "async" and "await". Let me introduce these two keywords for the time being.
Read Disclaimer Notice
The History
Before the introduction of these features, we generally rely on traditional approaches of creating Thread from a ThreadPool and consuming a method which needed to be asynchronously called for. Again when the Thread finishes, or during the lifetime of the Thread we need callbacks to notify UI after a while, so that the user gets an impression that the background is updating the system. This breaks the way we write or the way we think of programming. The programmer needs to create the program to device in such a way that it handles continuity of the application by writing custom callbacks to the system or even use Dispatcher to update the UI thread from background. Thus the code becomes more and more complex as we add up more functionalities to the system.
The Present
In this PDC, Anders Hejlsberg introduces two new investments on C# which is probably now still in CTP to allow asynchronous calls to the applications very simple and easy to handle. The two keyword which were introduced recently are "async" and "await". Let me introduce these two keywords for the time being.
Read Disclaimer Notice
Create Enumeration as Bit Flags
Posted by
Abhishek Sur
on Monday, October 25, 2010
Labels:
.NET,
C#,
CodeProject
11
comments
Enumeration is one the important feature of C#. Enumeration allows you to name a variable to make sense for the program. It is in general impossible to understand numeric status values when there is more than two values for a single state of object. In such a case we give a logical name for the numeric integer and use the Logical name of it to call that particular state.
For instance :
Say you want to define the nature of a person.
Hmm... Putting it further, Enum might also come very handy when you want to use a combination of the same. Say for instance, a person can be both Logical and Caring. Now how could you define this? Do you need to define enumeration values for each of them ? I guess that would not be a good choice either.
Flags attribute in Enum plays a vital role if you want to use the values of an enumeration in combinations. So that each combination of enumeration values are mutually exclusive and does not overlap with another value of Enum. For bit fields, it is very easy and yet very handy to use Flags attribute rather than using your own logic to define the flags yourself.
Read Disclaimer Notice
For instance :
Say you want to define the nature of a person.
public enum Character { Caring, Honest, Loving, Desparate, Obedient, Logical, Practical }You might name these characteristics using numeric values, say you have Honest as 1, Loving as 2 etc. But it would be more logical to use an Enum instead.
Hmm... Putting it further, Enum might also come very handy when you want to use a combination of the same. Say for instance, a person can be both Logical and Caring. Now how could you define this? Do you need to define enumeration values for each of them ? I guess that would not be a good choice either.
Flags attribute in Enum plays a vital role if you want to use the values of an enumeration in combinations. So that each combination of enumeration values are mutually exclusive and does not overlap with another value of Enum. For bit fields, it is very easy and yet very handy to use Flags attribute rather than using your own logic to define the flags yourself.
Read Disclaimer Notice
DLR using Reflection.Emit (In Depth) Part 2
Posted by
Abhishek Sur
on Sunday, October 24, 2010
Labels:
.NET,
.NET 4.0,
C#,
DLR,
Reflection
2
comments
In my previous post, I was discussing how you could create your own assembly at runtime or rather how you could compile an assembly type dynamically using Reflection.Emit. In this post I will take it further by giving away a number of examples for your better understanding how to build your own custom types dynamically during runtime. I will also try to cover up a portion of MSIL concepts so that you could unleash the power of MSIL easily in your application.
The Basics
To build a dynamic type, the most important thing that we have discussed already are Builder classes. The BCL exposes a number of Builder classes that enables us to generate MSIL code dynamically during runtime and hence you can compile the same to produce the output.
From the above figure I have put some of the most important Builder classes marked in Red. But ultimately, you need instructions to run for your application. To write your IL Expression, Reflection.Emit provides a class call ILGenerator. ILGenerator (marked in blue) enables you to write your IL for a method or property. OpCodes are Operation code that determined Computer instructions. So while writing your instructions you need to pass your OpCodes and generate the instruction set for the Method.
Now going further with our example, let me demonstrate the code one by one so that you could easily build your own Code generator.
Implement IBuilder for your Assembly
Lets move to the actual implementation of IBuilder interface. As per our discussion, IBuilder contains 4 members, Sum, Divide, Multiply & substract.
Download Sample Application - 66 KB
Read Disclaimer Notice
The Basics
To build a dynamic type, the most important thing that we have discussed already are Builder classes. The BCL exposes a number of Builder classes that enables us to generate MSIL code dynamically during runtime and hence you can compile the same to produce the output.
From the above figure I have put some of the most important Builder classes marked in Red. But ultimately, you need instructions to run for your application. To write your IL Expression, Reflection.Emit provides a class call ILGenerator. ILGenerator (marked in blue) enables you to write your IL for a method or property. OpCodes are Operation code that determined Computer instructions. So while writing your instructions you need to pass your OpCodes and generate the instruction set for the Method.
Now going further with our example, let me demonstrate the code one by one so that you could easily build your own Code generator.
Implement IBuilder for your Assembly
Lets move to the actual implementation of IBuilder interface. As per our discussion, IBuilder contains 4 members, Sum, Divide, Multiply & substract.
public interface IBuilder { float Sum(int firstnum, int secondnum); float Substract(int firstnum, int secondnum); float Multiply(int firstnum, int secondnum); float Divide(int firstnum, int secondnum); }
Download Sample Application - 66 KB
Read Disclaimer Notice
DLR using Reflection.Emit (In Depth) Part 1
Posted by
Abhishek Sur
on Saturday, October 23, 2010
Labels:
C#,
DLR,
Reflection
1 comments
I love C#, I Love .NET. Do you agree ? Hmm, But I mean it :)
Well, lets put it in other words, " The more I see the framework, the more I discover in .NET". Yes, after putting my efforts with Reflection classes, I thought I could make some research on code generation. I took the CodeDom being the best alternative to generate code. Sooner or later, I found out, CodeDom actually allows you to build your assembly but it is does not allow you to dynamically compile a part of the assembly at runtime, but rather it invokes the compiler to do that. So rather than CodeDom, I thought there must be something else which fruits my needs.
Next I found out one, using Expression Trees. If you are already following me, I think you know, few days back I have already written about Expression Trees and Lamda Decomposition. So it is not a good time to recap the same. Later on, I did some research on MSIL, and found it worth learning. If you are going to grow with .NET, it would be your added advantage if you know about MSIL. Hence I started looking at the MSIL. Finally I found out a number of classes which might help you to build a Type dynamically. Let me share the entire thing with you.
Introduction
Reflection.Emit like CodeDom allows you to build your custom assembly and provides you a number of Builder classes which might be compiled during Runtime, and hence invoke DLR capabilities of C#. The library also exposes one ILGenerator which might be used later to produce the actual MSIL by putting efforts to emit Operation codes. So finally after you write your OpCodes correctly, you could easily able to compile the type dynamically during runtime. In this post, I would use ILDASM to see the IL generated from our own class, that I define, and later on I would try to build the same class dynamically.
What is Reflection ?
If you are struck with Reflection, then you need to really gear yourself a bit to go further. Let me give a brief explanation of Reflection. Reflection is actually a technique to read a managed dll which is not being referenced from the application and call its types. In other words, it is a mechanism to discover the types and call its properties at runtime. Say for instance, you have an external dll which writes logger information and sends to the server. In that case, you have two options.
To know more try Reflection Overview
What is Reflection.Emit ?
Being a part of Reflection, Reflection.Emit namespace list you a number of classes which you can use to build your type. As I have already told you, Reflection.Emit actually provides you some Builder classes like AssemblyBuilder, ModuleBuilder, ConstructorBuilder, MethodBuilder, EventBuilder, PropertyBuilder etc. which allows you to build your IL dynamically during run time. The ILGenerator provides you the capabilities to generate your IL and place the same for a method. Generally, it is very rare that a developer need these capabilities to generate an assembly at runtime, but it is great to find these capabilities present in the framework.
Now lets see what is required to build an assembly.
Read Disclaimer Notice
Well, lets put it in other words, " The more I see the framework, the more I discover in .NET". Yes, after putting my efforts with Reflection classes, I thought I could make some research on code generation. I took the CodeDom being the best alternative to generate code. Sooner or later, I found out, CodeDom actually allows you to build your assembly but it is does not allow you to dynamically compile a part of the assembly at runtime, but rather it invokes the compiler to do that. So rather than CodeDom, I thought there must be something else which fruits my needs.
Next I found out one, using Expression Trees. If you are already following me, I think you know, few days back I have already written about Expression Trees and Lamda Decomposition. So it is not a good time to recap the same. Later on, I did some research on MSIL, and found it worth learning. If you are going to grow with .NET, it would be your added advantage if you know about MSIL. Hence I started looking at the MSIL. Finally I found out a number of classes which might help you to build a Type dynamically. Let me share the entire thing with you.
Introduction
Reflection.Emit like CodeDom allows you to build your custom assembly and provides you a number of Builder classes which might be compiled during Runtime, and hence invoke DLR capabilities of C#. The library also exposes one ILGenerator which might be used later to produce the actual MSIL by putting efforts to emit Operation codes. So finally after you write your OpCodes correctly, you could easily able to compile the type dynamically during runtime. In this post, I would use ILDASM to see the IL generated from our own class, that I define, and later on I would try to build the same class dynamically.
What is Reflection ?
If you are struck with Reflection, then you need to really gear yourself a bit to go further. Let me give a brief explanation of Reflection. Reflection is actually a technique to read a managed dll which is not being referenced from the application and call its types. In other words, it is a mechanism to discover the types and call its properties at runtime. Say for instance, you have an external dll which writes logger information and sends to the server. In that case, you have two options.
- You refer to the assembly directly and call its methods.
- You use Reflection to load the assembly and call it using interfaces.
To know more try Reflection Overview
What is Reflection.Emit ?
Being a part of Reflection, Reflection.Emit namespace list you a number of classes which you can use to build your type. As I have already told you, Reflection.Emit actually provides you some Builder classes like AssemblyBuilder, ModuleBuilder, ConstructorBuilder, MethodBuilder, EventBuilder, PropertyBuilder etc. which allows you to build your IL dynamically during run time. The ILGenerator provides you the capabilities to generate your IL and place the same for a method. Generally, it is very rare that a developer need these capabilities to generate an assembly at runtime, but it is great to find these capabilities present in the framework.
Now lets see what is required to build an assembly.
Read Disclaimer Notice
Hidden Facts of C# Structures in terms of MSIL
Posted by
Abhishek Sur
on Saturday, October 16, 2010
Labels:
.NET,
.NET 4.0,
C#,
CodeProject,
Memory Allocation
4
comments
Did you ever thought of a program without using a single structure declared in it ? Never thought so na? I did that already but found it would be impossible to do that, at least it does not make sense as the whole program is internally running on messages passed from one module to another in terms of structures.
Well, well hold on. If you are thinking that I am talking about custom structures, you are then gone too far around. Actually I am just talking about declaration of ValueTypes. Yes, if you want to do a calculation, by any means you need an integer, and System.Int32 is actually a structure. So for my own program which runs without a single ValueType declared in it turns out to be a sequence of Print statements. Does'nt makes sense huh! Really, even I thought so. Thus I found it is meaningless to have a program which just calls a few library methods (taking away the fact that library methods can also create ValueType inside it) and prints arbitrary strings on the output window.
Please Note : As struct is actually an unit of ValueType, I have used it synonymously in the post.
So what makes us use Structures so often?
The first and foremost reason why we use structures is that
Read Disclaimer Notice
Well, well hold on. If you are thinking that I am talking about custom structures, you are then gone too far around. Actually I am just talking about declaration of ValueTypes. Yes, if you want to do a calculation, by any means you need an integer, and System.Int32 is actually a structure. So for my own program which runs without a single ValueType declared in it turns out to be a sequence of Print statements. Does'nt makes sense huh! Really, even I thought so. Thus I found it is meaningless to have a program which just calls a few library methods (taking away the fact that library methods can also create ValueType inside it) and prints arbitrary strings on the output window.
Please Note : As struct is actually an unit of ValueType, I have used it synonymously in the post.
So what makes us use Structures so often?
The first and foremost reason why we use structures is that
- They are very simple with fast accessibility.
- It is also created on Thread local stack, which makes it available only to the current scope and destroyed automatically after the call is returned. It doesn't need any external invisible body to clear up the memory allocated henceforth.
- Most of the primitives have already been declared as struct.
- In case of struct we are dealing with the object itself, rather than with the reference of it.
- Implicit and explicit operators work great with struct and most of them are included already to the API.
Read Disclaimer Notice
ASP.NET 4.0 In - Depth Session PART 2 On 10th OCT
Posted by
Abhishek Sur
on Tuesday, October 5, 2010
Labels:
.NET 4.0,
ASP.NET 4.0,
Online Session
3
comments
Guys, after completing the Part 1 of the Session, its time to go beyond it and show you a few more advanced concepts from ASP.NET 4.0. The remaining part of the Session includes :
I will present this Session with Abhijit Jana on 10th October (the next Sunday) from 2 P.M IST. Please visit http://www.dotnetfunda.com/misc/onlinesession.aspx for more details.
If you missed out the Previous Session, you can follow the link to download PPT, the Video and the Source codes :
Download PPT, Sample Code & Video1
Download PPT, Sample Code & Video1
I hope the next session would be as good as this one. Nominate yourself for the same.(You need to register before you nominate yourself) Thank you all for your support.
Be there.
Note : If you have already nominated for the Session you do not need to Renominate yourself. Read Disclaimer Notice
Grayed Portion is already covered in PART 1 |
- State Management Enhancements
- Client side Enhancements
- Deployment
- Overview of Dynamic Data
I will present this Session with Abhijit Jana on 10th October (the next Sunday) from 2 P.M IST. Please visit http://www.dotnetfunda.com/misc/onlinesession.aspx for more details.
If you missed out the Previous Session, you can follow the link to download PPT, the Video and the Source codes :
Download PPT, Sample Code & Video1
Download PPT, Sample Code & Video1
I hope the next session would be as good as this one. Nominate yourself for the same.(You need to register before you nominate yourself) Thank you all for your support.
Be there.
Note : If you have already nominated for the Session you do not need to Renominate yourself. Read Disclaimer Notice
Lazy Load XAML content from External File and Vice Versa
Posted by
Abhishek Sur
on Monday, October 4, 2010
Labels:
.NET 4.0,
CodeProject,
WPF
2
comments
XAML is the most flexible language built ever. More I see XAML, more I know about it. Today while tweaking around with XAML code, I found XAML could be loaded dynamically from any XML string. That means if you have a xaml in a xml file you can probably load the part of the XAML into your ContentControl or to any control element you want and the UI will appear instantly.
Once you compile a XAML it produces BAML. BAML is in binary format for the XML, so if you can pass a BAML into the UI separately somehow during runtime,you would be seeing the content instantly in the Window. In this post I am going to discuss how easily you could load a Dynamic content of XAML file into a normal WPF ContentControl just like what we do for normal htmls.
What is XamlReader and XamlWriter?
If you look into the implementation of these classes you could wonder how flexible these are. They are highly capable of parsing the whole content of the file. It uses a XAMLDictionary which holds all the XAML elements that a XAML can see. The Reader parses the Xml content very cautiously to ensure it makes the XAML file to contain no reference of outside. Thus the class is used to Refactor the XAML from outside and hence allows you to put the content anywhere such that everything will be applied on that instantly.
XamlReader exposes methods like Load / Parse which allows you to parse a file content into BAML. Hence each of them returns an binary object which you can put into the Content.
Download Sample Application - 56 KB
Read Disclaimer Notice
Once you compile a XAML it produces BAML. BAML is in binary format for the XML, so if you can pass a BAML into the UI separately somehow during runtime,you would be seeing the content instantly in the Window. In this post I am going to discuss how easily you could load a Dynamic content of XAML file into a normal WPF ContentControl just like what we do for normal htmls.
What is XamlReader and XamlWriter?
If you look into the implementation of these classes you could wonder how flexible these are. They are highly capable of parsing the whole content of the file. It uses a XAMLDictionary which holds all the XAML elements that a XAML can see. The Reader parses the Xml content very cautiously to ensure it makes the XAML file to contain no reference of outside. Thus the class is used to Refactor the XAML from outside and hence allows you to put the content anywhere such that everything will be applied on that instantly.
XamlReader exposes methods like Load / Parse which allows you to parse a file content into BAML. Hence each of them returns an binary object which you can put into the Content.
Download Sample Application - 56 KB
Read Disclaimer Notice
Building a CRUD in RESTful Services of WCF
Posted by
Abhishek Sur
on Sunday, October 3, 2010
Labels:
.NET 4.0,
C#,
WCF
8
comments
WCF is being popular day by day. Many of us is building services using WCF and want other application made on different architecture to communicate or inter operate with each other. REST or Representational State Transfer is a Design Pattern to build a service so that it works the same way as service works but it will eventually use Web Request - Response mechanism to communicate between clients rather than using SOAP messages. In this post, I will give you a step by step approach how you could build your own WCF service using REST.
What is REST ?
REST abbreviated as Representational State Transfer is coined by Roy Fielding is a Design pattern for Networked system. In this pattern the Client invokes a Web request using an URL, so the basic of REST pattern is to generate unique URI to represent data.
If you look into Roy Fielding's quote on REST it says :
"Representational State Transfer is intended to evoke an image of how a well-designed Web application behaves: a network of web pages (a virtual state-machine), where the user progresses through an application by selecting links (state transitions), resulting in the next page (representing the next state of the application) being transferred to the user and rendered for their use."
That means REST is intended for Web applications where the browser invokes each request by specifying unique Uri.
Download Sample Application - 200KB
Read Disclaimer Notice
What is REST ?
REST abbreviated as Representational State Transfer is coined by Roy Fielding is a Design pattern for Networked system. In this pattern the Client invokes a Web request using an URL, so the basic of REST pattern is to generate unique URI to represent data.
If you look into Roy Fielding's quote on REST it says :
"Representational State Transfer is intended to evoke an image of how a well-designed Web application behaves: a network of web pages (a virtual state-machine), where the user progresses through an application by selecting links (state transitions), resulting in the next page (representing the next state of the application) being transferred to the user and rendered for their use."
That means REST is intended for Web applications where the browser invokes each request by specifying unique Uri.
REST Based Services |
Download Sample Application - 200KB
Read Disclaimer Notice
5 things I would like to see in C# 5.0
Posted by
Abhishek Sur
on Wednesday, September 29, 2010
Labels:
.NET,
.NET 3.5,
.NET 4.0,
C#,
CodeProject
12
comments
C# 4.0 is out with a lots of new specs this year. Few of them are really good, like the support of Task level Parallelism, Dynamic behavior of objects etc. So C# is very rich now in all respect. But this is not the end of everything. As I did introduced few old but Unknown C# topics, I would also love to explore new things that comes up with C#. So lets discuss a few things which I would love to have.
There is already a buzz around on C# 5.0 specifications in forums. A notable discussion around should be some threads like
What features you want to see in C# 5.0
C# language speculation 4.5 /5.0
etc. These community links are really great where a lots of people voted for new language specs, while quite a few things are already would be in process like :
Binding Operators
Binding Operators may be C# 5.0. The support for Binding on .net objects are awesome. I came to know this from Chris on his post where he speaks how Binding operators looks like long ago. The delphi assignment operator := is now been used in this purpose. :=: would be used for two way binding. Thus
means there would be two way binding between the properties so that when ComboBox1.Text changes its Text it will automatically change the value of Textbox1.Text.
Binding is actually not a new thing to the system. In WPF we already have Binding in place, but for that we need the properties to have implemented from INotifyPropertyChanged. So it is not in the language, the WPF objects are doing this internally by handling the PropertyChanged event on the object. But if that is included as an operator it would be a cool feature to add on.
Read Disclaimer Notice
There is already a buzz around on C# 5.0 specifications in forums. A notable discussion around should be some threads like
What features you want to see in C# 5.0
C# language speculation 4.5 /5.0
etc. These community links are really great where a lots of people voted for new language specs, while quite a few things are already would be in process like :
Binding Operators
Binding Operators may be C# 5.0. The support for Binding on .net objects are awesome. I came to know this from Chris on his post where he speaks how Binding operators looks like long ago. The delphi assignment operator := is now been used in this purpose. :=: would be used for two way binding. Thus
comboBox1.Text :=: textBox1.Text;
means there would be two way binding between the properties so that when ComboBox1.Text changes its Text it will automatically change the value of Textbox1.Text.
Binding is actually not a new thing to the system. In WPF we already have Binding in place, but for that we need the properties to have implemented from INotifyPropertyChanged. So it is not in the language, the WPF objects are doing this internally by handling the PropertyChanged event on the object. But if that is included as an operator it would be a cool feature to add on.
Read Disclaimer Notice
WPF Tutorial : Styles, Triggers and Animations : 7
Posted by
Abhishek Sur
on Monday, September 27, 2010
Labels:
.NET 3.5,
.NET 4.0,
C#,
WPF
0
comments
Introduction
Perhaps the most interesting and most important feature for any WPF application is Styling. Styling means defining styles for controls, and store in reusable ResourceDictionaries and hence forth, it could be used later on by calling its name. Styles in WPF could be well compared with CSS styles. They are both similar in most of the cases, while the former extends the feature allowing most of the features which WPF have. In this article I will discuss mostly how you could use Style in your WPF application to enhance the Rich experience of your UI.
Style
WPF exposes a property Style for every Control. If you look into the object Hierarchy, the Style is basically a property which exposes an object of Style in FrameworkElement. So each object can associate it and define custom setters to manipulate the basic look and feel of a control.
Clearly, the above diagram shows the association of Style in FrameworkElement and from the object hierarchy every control somehow inherits from FrameworkElement and hence style will be available to it. Style is also a WPF object which is inherited form DispatcherObject which helps in setting different properties of your UI Element.
Before we move further into Styles lets talk about Themes. Theme is totally different from Styles. Themes are defined at OS level, or more precisely a Theme can take part of delivering styles all over the Desktop while Styles are restricted to the contextual area of a WPF window. WPF are capable of retrieving the color scheme which is defined in OS level. Say for instance, if you do not define style for your application, the elements in the screen will automatically get styles from external environment. Say for instance, in XP if you change the theme to something else you would see that the buttons, TextBox on your WPF window will change its color instantly. You can even set the Theme which the application would use programmatically from your code.
Every control defines a ControlTemplate. A ControlTemplate defines the overall structure of the control. As I have already told you, say for instance you have a Button. Button is a control that is made up of more than one control. It would have a ContentPresenter which writes the Text over the control, it would have a Rectangle which keeps the boundary of the Button etc. So Template is a special property associated with a Control which specifies how the control will look like structurally. We can easily define our Template and change the overall structure of a control.
ControlTemplate defines the structure of the Control. It means say for instance, you define the ControlTemplate for a ComboBox. So from ControlTemplate you can easily change the Button associated with the ComboBox which opens the DropDown, you can change the structure of the TextBox, the Popup etc. So ControlTemplate allows you to change the overall structure of the Control.
Click To Read Entire Article
Read Disclaimer Notice
Perhaps the most interesting and most important feature for any WPF application is Styling. Styling means defining styles for controls, and store in reusable ResourceDictionaries and hence forth, it could be used later on by calling its name. Styles in WPF could be well compared with CSS styles. They are both similar in most of the cases, while the former extends the feature allowing most of the features which WPF have. In this article I will discuss mostly how you could use Style in your WPF application to enhance the Rich experience of your UI.
Style
WPF exposes a property Style for every Control. If you look into the object Hierarchy, the Style is basically a property which exposes an object of Style in FrameworkElement. So each object can associate it and define custom setters to manipulate the basic look and feel of a control.
Clearly, the above diagram shows the association of Style in FrameworkElement and from the object hierarchy every control somehow inherits from FrameworkElement and hence style will be available to it. Style is also a WPF object which is inherited form DispatcherObject which helps in setting different properties of your UI Element.
How Style differs from Theme ?
Before we move further into Styles lets talk about Themes. Theme is totally different from Styles. Themes are defined at OS level, or more precisely a Theme can take part of delivering styles all over the Desktop while Styles are restricted to the contextual area of a WPF window. WPF are capable of retrieving the color scheme which is defined in OS level. Say for instance, if you do not define style for your application, the elements in the screen will automatically get styles from external environment. Say for instance, in XP if you change the theme to something else you would see that the buttons, TextBox on your WPF window will change its color instantly. You can even set the Theme which the application would use programmatically from your code.
What about Templates ?
Every control defines a ControlTemplate. A ControlTemplate defines the overall structure of the control. As I have already told you, say for instance you have a Button. Button is a control that is made up of more than one control. It would have a ContentPresenter which writes the Text over the control, it would have a Rectangle which keeps the boundary of the Button etc. So Template is a special property associated with a Control which specifies how the control will look like structurally. We can easily define our Template and change the overall structure of a control.
Templates are basically of 2 types :
- ControlTemplate
- DataTemplate
ControlTemplate defines the structure of the Control. It means say for instance, you define the ControlTemplate for a ComboBox. So from ControlTemplate you can easily change the Button associated with the ComboBox which opens the DropDown, you can change the structure of the TextBox, the Popup etc. So ControlTemplate allows you to change the overall structure of the Control.
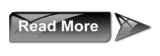
Progress Streamed File download and Upload with Resume facility
Posted by
Abhishek Sur
on Saturday, September 25, 2010
Labels:
.NET 4.0,
CodeProject,
WCF,
WPF
1 comments
WCF allows you to send Byte streams through the communication channel or even allows you to implement a service that might take the stream start location from the service call and send the stream from a certain location. It allows you to use normal HttpBinding to support streams which is capable download or upload in connected Client - Server architecture. Hence there will be not timeouts for sending or receiving large files. In this article I will show you how you could use Streaming to ensure your files are downloaded or uploaded correctly.
Once I read Demitris solution for File upload and download it made me very easy to adjust the code and enhance it further with more flexibilities.
Lets first create the service step by step :
Service
- Create a Service Application and name it as WCFCommunicationService
- The first thing that you need to do in order to create a service is ServiceContract. So once you create a Service Library, you need to delete the existing Service1 and IService1 files from the solution and add a new Interface.
- Create Two method, one for FileDownload and another for FileUpload. Lets see how the service definition looks like :
[ServiceContract] public interface IFileTransferLibrary { [OperationContract()] void UploadFile(ResponseFile request); [OperationContract] ResponseFile DownloadFile(RequestFile request); }
The Contract defines the methods that are exposed to outside. The ServiceContract defines the interface which will have the members which are available to outside. The OperationContract identifies the exposed members. In the above contract the IFileTransferLibrary has two methods, UploadFile which takes an object of ResponseFile and DownloadFile which returns an object of ResponseFile.
[MessageContract] public class ResponseFile : IDisposable { [MessageHeader] public string FileName; [MessageHeader] public long Length; [MessageBodyMember] public System.IO.Stream FileByteStream; [MessageHeader] public long byteStart = 0; public void Dispose() { if (FileByteStream != null) { FileByteStream.Close(); FileByteStream = null; } } } [MessageContract] public class RequestFile { [MessageBodyMember] public string FileName; [MessageBodyMember] public long byteStart = 0; }
If you see the classes RequestFile and ResponseFile, you will see that I have used MessageContract instead of DataContract for my complex types. It is important. AS I am going to send the data streamed to the client, I need to send the data into packets. DataContract will send the whole object at a time while Messagecontract sends them into small Messages.It is also important to note that I have used IDisposable for ResponseFile to ensure that the Stream is closed whenever the connection is terminated.
Another important note is you need to use Stream as MessageBodyMember. The stream data will always transferred in MessageBody and you cannot add any other member into it. Thus you can see I have put all other members as MessageHeader.
For Both UploadFile and DownloadFile the Stream is taken from the ResponseFile object. The byteStart element of RequestFile ensures from where the the downloading should start and hence helps in Resuming a half downloaded file. Lets see how to implement this :
public ResponseFile DownloadFile(RequestFile request) { ResponseFile result = new ResponseFile(); FileStream stream = this.GetFileStream(Path.GetFullPath(request.FileName)); stream.Seek(request.byteStart, SeekOrigin.Begin); result.FileName = request.FileName; result.Length = stream.Length; result.FileByteStream = stream; return result; } private FileStream GetFileStream(string filePath) { FileInfo fileInfo = new FileInfo(filePath); if (!fileInfo.Exists) throw new FileNotFoundException("File not found"); return new FileStream(filePath, FileMode.Open, FileAccess.Read); } public void UploadFile(ResponseFile request) { string filePath = Path.GetFullPath(request.FileName); int chunkSize = 2048; byte[] buffer = new byte[chunkSize]; using (FileStream stream = new FileStream(filePath, FileMode.Append, FileAccess.Write)) { do { int readbyte = request.FileByteStream.Read(buffer, 0, chunkSize); if (readbyte == 0) break; stream.Write(buffer, 0, readbyte); } while (true); stream.Close(); } }
If you see the code, you can see how I have used byteStart to start sending Bytes for the file. This ensures the file download to have resume facility. You can also implement the same for UploadFile too.
Download FileTransfer Sample - 161KB
Read Disclaimer Notice
Online Session on ASP.NET
Posted by
Abhishek Sur
on Sunday, September 19, 2010
Labels:
.NET 4.0,
ASP.NET 4.0,
dotnetfunda.com
2
comments
I have just covered an Online session on "ASP.NET 4.0 in depth features" with Abhijit Jana for DotNetFunda.Com. If you were already there for the session, I hearty congratulate you people for your co-operation and also like you to join us in the concluding part of the session which will be declared later in the day.
In the session :
Things that we have covered already :
You can download Sample Applications that I showed :
1. Routing Demo Application
2. Meta Tags and Parmanent Redirections
The sample video :
Download PPT, Sample Code & Video1
Download PPT, Sample Code & Video2
You can download the sample application and also run the PPT and Video from the link above. (Let me know if the link goes down.)
For reference Reading :
ASP.NET 4.0 WhitePapers
Thanks for being a part of the session.
Hope to see you soon. Read Disclaimer Notice
In the session :
Things that we have covered already :
- Basic Introduction of ASP.NET 4.0 Features
- Visual Studio Enhancement Session
- Server Control Enhancements
- Search Engine Optimization
You can download Sample Applications that I showed :
1. Routing Demo Application
2. Meta Tags and Parmanent Redirections
The sample video :
Download PPT, Sample Code & Video1
Download PPT, Sample Code & Video2
You can download the sample application and also run the PPT and Video from the link above. (Let me know if the link goes down.)
The Grayed items are already discussed, while others will be taken in the next session which will be announced shortly. |
For reference Reading :
ASP.NET 4.0 WhitePapers
Thanks for being a part of the session.
Hope to see you soon. Read Disclaimer Notice
How to create a WCF service without Visual Studio
Posted by
Abhishek Sur
on Wednesday, September 15, 2010
Labels:
.NET,
.NET 3.5,
C#,
CodeProject,
WCF
3
comments
WCF is the first step in building a truly service oriented application for you. It is the most important when working with distributed architecture. The sophisticated design of WCF made me think of using it always when I need any Web service to be installed in the server. Visual Studio is capable of creating its own configuration settings that helps in developing our application with ease. But what if you don’t have Visual Studio? In this post I am going to implement one of the most basic WCF service without using Visual Studio and show you how to interact with the service. Lets do it step by step:
Server Side
Steps to create the Service definition (Contract):
Steps to host the service (Address , Binding):
To host the service in the server you need to know three inputs:
Read Disclaimer Notice
Server Side
Steps to create the Service definition (Contract):
- Open Notepad and add namespace System and System.ServiceModel. We need ServiceModel to specify a WCF service
- For WCF service we need to create an interface which will act as a proxy to the client. From the client, we need to replicate the proxy object and build the same interface again. After we declare the Interface we mark the Interface with ServiceContract, and the method to be exposed to outside using OperationContract.
- Next we create a concrete class for the same to define the class implementing the interface.
[ServiceContract] public interface IOperationSimpleWCF { [OperationContract] string MySimpleMethod(string inputText); } public class OperationSampleWCF : IOperationSimpleWCF { public string MySimpleMethod(string inputText) { Console.WriteLine("Message Received : {0}", inputText); Console.WriteLine(OperationContext.Current.RequestContext.RequestMessage.ToString()); return string.Format("Message from Server {0}", inputText); } }
Steps to host the service (Address , Binding):
To host the service in the server you need to know three inputs:
- Binding : This indicates how the service will be hosted. For basic soap operation with no security we need HttpBinding. We can also use other bindings as well.
- Address : Represents the location to host the service. When the service is hosted you can specify the qualified service path where the service will be hosted.
- Host the service using ServiceHost and Open the connection.
Read Disclaimer Notice
Partial Methods - An Uncommon Note
Posted by
Abhishek Sur
on Tuesday, September 14, 2010
Labels:
.NET 3.5,
C#,
CodeProject
2
comments
I think most of the people working in C# must know how Partial classes work. Partial classes allows you to divide your own class in one or more files but during runtime the files would be merged to produce a single coherent object. By this way the classes could be made very small and also easily maintainable.
The most important part of a Partial class is with designers. While you work in Visual Studio, you must have found a portion of code that is generated automatically by the designer. Designers creates a class which keeps on refreshing itself when certain modifications are made in the Designer itself. Partial classes allows you to create an alternate implementation of the same class so that the code you add to the class does not goes out when the Designer is refreshed.
Partial Methods are another new addition to .NET languages that allows you to declare / define the same method more than once. Generally Microsoft introduced the concept of Partial method to deal with Designer generated code and to allow reserving the name of a method that one needed to be implemented later in original classes. By this way, the Designer will not produce any warning without implementing the actual method, but will allow future developers to implement or write their own logic on the same method that is defined.
Let us suppose the MyPartialClass is generated by the designer. It declares the method MyPartialMethod which I have not been defined yet.
In another implementation of MyPartialClass, I have defined the method MyPartialMethod which holds the actual implementation of the code. So if I create an object of MyPartialClass, it will hold both method MyNormalMethod and MyPartialMethod and when it is called it calls appropriately.
Another thing to note, the implementation of Partial method is not mandatory. If you do not define the partial method in another partial class, it will eventually be eliminated completely in runtime IL.
Limitations of a Partial Method :
Since partial method is created to generate methods in designer, most of the designer highly using partial methods. Even though it is very rarely used in real life, it would be worth if you know about it.
For further reference:
C# 3.0 Partial Method - What Why and How
Thank you for reading my post.
The most important part of a Partial class is with designers. While you work in Visual Studio, you must have found a portion of code that is generated automatically by the designer. Designers creates a class which keeps on refreshing itself when certain modifications are made in the Designer itself. Partial classes allows you to create an alternate implementation of the same class so that the code you add to the class does not goes out when the Designer is refreshed.
Partial Methods are another new addition to .NET languages that allows you to declare / define the same method more than once. Generally Microsoft introduced the concept of Partial method to deal with Designer generated code and to allow reserving the name of a method that one needed to be implemented later in original classes. By this way, the Designer will not produce any warning without implementing the actual method, but will allow future developers to implement or write their own logic on the same method that is defined.
partial class MyPartialClass { public void MyNormalMethod() { Console.WriteLine("Inside first Normal Method"); } partial void MyPartialMethod(); //It is declared and will be defined later in another partial class }
Let us suppose the MyPartialClass is generated by the designer. It declares the method MyPartialMethod which I have not been defined yet.
partial class MyPartialClass { //public void MyNormalMethod() //{ // Console.WriteLine("Inside second Normal Method"); //} partial void MyPartialMethod() { Console.WriteLine("Inside second Partial Method"); } }
In another implementation of MyPartialClass, I have defined the method MyPartialMethod which holds the actual implementation of the code. So if I create an object of MyPartialClass, it will hold both method MyNormalMethod and MyPartialMethod and when it is called it calls appropriately.
Another thing to note, the implementation of Partial method is not mandatory. If you do not define the partial method in another partial class, it will eventually be eliminated completely in runtime IL.
Limitations of a Partial Method :
- Partial Method is by default private. Hence you can only call a partial method from within the partial class.
partial class MyPartialClass { public void MyNormalMethod() { Console.WriteLine("Inside first Normal Method"); this.MyPartialMethod(); } partial void MyPartialMethod(); //It is declared and will be defined later in another partial class }
Thus even if you do not declare the method MyPartialMethod, you can even call it from within the class. During runtime, CLR will automatically call if it is implemented or will eliminate the call completely from the system.
- Partial Method needed to be declared inside a Partial class. A partial class is only capable of redefining the part later in another file. So it is essential to mark the class as partial as well when it have a partial method.
- Cannot be marked as extern.
- Must have a void return type and also cannot use out parameter as argument.
- Partial method does not allow to write as virtual, sealed, new, override or extern.
Since partial method is created to generate methods in designer, most of the designer highly using partial methods. Even though it is very rarely used in real life, it would be worth if you know about it.
For further reference:
C# 3.0 Partial Method - What Why and How
Thank you for reading my post.
Marble Diagrams and Rx
Posted by
Abhishek Sur
on Sunday, September 12, 2010
Labels:
.NET 4.0,
C#,
CodeProject,
IObservable. Rx
3
comments
Today I am going to discuss how to draw Marble Diagrams. I just came across with it in Reactive Framework. In Channel 9 videos, Jeffrey Van Gogh shows how to get started with Rx. Ever since I look deep into it, I find it very interesting, and perhaps a new way of thinking or understanding for Asynchronous programming structure. Reactive Framework introduces a few operators, which you might apply to an Observable to get the resultant observable. The way to represent each of these attributes can be done using Marble Diagrams.
Before you start dealing with Reactive Framework, it is good to start with IObservable and IObserver. I have already introduced with these two interfaces which are the building block for the Reactive Framework. You can read these articles before going through with Marble Diagrams.
How to create Marble Diagram.
Marble diagram is basically a way to represent the Rx operations. You may think a marble diagram to be a pictorial representation of different operators that is defined with Reactive Framework. Lets look how to create a Marble Diagram :
So in the sample image you can see we measure Time in X-Axis while operations on Y-axis. Say for instance, you have an Observable which is represented by the first horizontal line. The small circles represents OnNext calls of IObserver. If you know already read about Observable and Observer, you might already know that each Observer has OnNext method, which gets invoked whenever the observer changes its state. Hence, in our case the observer invokes a function f() to get to the new State. The | represents the OnComplete for the Observer. So after OnComplete, the Observer will stop observing states.
We represents OnError using X in a marble diagram. In case of an Observable, OnError and OnComplete produces the end point for the observer. So Here after executing the two OnNext if the Observer encounters with an Exception, OnError gets invoked and the Observer will terminate.
So on the first image, we execute the function f() to get the new state with the Buttom Line. Now on, I am going to create a few Marble Diagrams, to make your understanding clear.
SelectMany
SelectMany is very easy to explain. Say you have two or more Observers. In this case, SelectMany will eventually invoke all the OnNext of each observer.
So if you have 3 observers, the SelectMany will produce Observer which aggregates all of them.
In case of an error, the final Observer will stop when first error of any Observer is encountered.
SkipUntil / SkipWhile
SkipWhile and SkipUntil works in opposite. Say for instance, you have two Observable. SkipUntil gives you OnNext for each of them until the OnNext from the other observer is received. On the contrary, SkipWhile will produce OnNext for the Observer while the OnNext from the second observer is Received. When the second observer is Complete or gives an error, the final observer will terminate in case of SkipWhile.
So in the above diagrams, you can see While will bypass all the values until the second observer receives an entry.
Further Reference
To learn the operators of Marble Diagrams, you can see the latest Videos on Channel 9. Here is a few more Operators :
I hope this will help you to understand the Marble Diagrams, and hope these will describe you some more operators of Rx Framework. Read Disclaimer Notice
Before you start dealing with Reactive Framework, it is good to start with IObservable and IObserver. I have already introduced with these two interfaces which are the building block for the Reactive Framework. You can read these articles before going through with Marble Diagrams.
How to create Marble Diagram.
Marble diagram is basically a way to represent the Rx operations. You may think a marble diagram to be a pictorial representation of different operators that is defined with Reactive Framework. Lets look how to create a Marble Diagram :
So in the sample image you can see we measure Time in X-Axis while operations on Y-axis. Say for instance, you have an Observable which is represented by the first horizontal line. The small circles represents OnNext calls of IObserver. If you know already read about Observable and Observer, you might already know that each Observer has OnNext method, which gets invoked whenever the observer changes its state. Hence, in our case the observer invokes a function f() to get to the new State. The | represents the OnComplete for the Observer. So after OnComplete, the Observer will stop observing states.
We represents OnError using X in a marble diagram. In case of an Observable, OnError and OnComplete produces the end point for the observer. So Here after executing the two OnNext if the Observer encounters with an Exception, OnError gets invoked and the Observer will terminate.
So on the first image, we execute the function f() to get the new state with the Buttom Line. Now on, I am going to create a few Marble Diagrams, to make your understanding clear.
SelectMany
SelectMany is very easy to explain. Say you have two or more Observers. In this case, SelectMany will eventually invoke all the OnNext of each observer.
So if you have 3 observers, the SelectMany will produce Observer which aggregates all of them.
int[] collection = { 2, 5, 5, 6, 2, 4 }; int[] collection2 = { 1, 4, 2, 1, 5, 6 }; var ob1 = Observable.ToObservable(collection); var ob2 = Observable.ToObservable(collection2); var ob3 = ob1.SelectMany(ob2); //var ob3 = ob1.Select(r => r + 2); var disp = ob3.Subscribe(r => Console.WriteLine("OnNext : {0}", r.ToString()), r => Console.WriteLine("Error : {0}", r.ToString()), () => Console.WriteLine("Completed")); disp.Dispose();So the Observer will select from each of the Observer and get you the output.
In case of an error, the final Observer will stop when first error of any Observer is encountered.
SkipUntil / SkipWhile
SkipWhile and SkipUntil works in opposite. Say for instance, you have two Observable. SkipUntil gives you OnNext for each of them until the OnNext from the other observer is received. On the contrary, SkipWhile will produce OnNext for the Observer while the OnNext from the second observer is Received. When the second observer is Complete or gives an error, the final observer will terminate in case of SkipWhile.
So in the above diagrams, you can see While will bypass all the values until the second observer receives an entry.
Further Reference
To learn the operators of Marble Diagrams, you can see the latest Videos on Channel 9. Here is a few more Operators :
I hope this will help you to understand the Marble Diagrams, and hope these will describe you some more operators of Rx Framework. Read Disclaimer Notice
Taskbar with Window Maximized and WindowState to None in WPF
Posted by
Abhishek Sur
on Tuesday, September 7, 2010
Labels:
.NET 3.5,
.NET 4.0,
C#,
CodeProject,
WPF
7
comments
Say you want your WPF application to have no Title bar, and will maximized to Full Screen, what you first think of. Its the most easiest to do.
Oh.. Hold on ..Hold on... As I say, it hides the whole screen, it means it even will not show up the Taskbar or any external gadgets applied to your desktop if it is not set to Always on Top attribute to it.
Yes, this is the problem that I faced recently, when people wanted me to show the TaskBar even though the application should be Maximized.
Is it a Bug ?
If I am not wrong, WPF builds application based on the Screen Resolution. It produces DPI independent pixels. If you specify it to be full screen, it first gets the Resolution of the screen, and draws the pixel based on its own algorithm. Hence, when you specify it to Maximized, it takes up the whole screen, as otherwise some portion of the screen will be hidden outside the range of the boundary.
When you restore a WPF screen, it will also recalculate the work area based on the distance between the resolution boundaries and resize itself accordingly. No do you think Microsoft should really have an alternative state which show up the Taskbar as it does with normal windows ? I think yes.
The Solution
As I needed to do this, I have just tried out few workarounds to this myself.
Following Lester's Blog on this issue, I have to use
The call to API WmGetMinMaxInfo gets you the size of Maximize window for the current desktop.
The call to GetMonitorInfo gets you a MONITORINFO object and if you see the code carefully it actually position the window in such a way that it absolutely resize itself to the height and width of the rectangular area.
To call this method, I can use SourceInitialized event which will eventually be called whenever the WindowState is modified.
Sounds good ?
Oh.. Lets give another easier solution that I have used. Its very simple.
Set properties for your window :
And go to your code and just resize your window based on PrimaryScreen width and height. Also make sure you do set the Left and Top of the window as well.
Making WindowState.Normal will ensure that the default behaviour of the window is overridden and also makes the Taskbar Reappear.
I have included my sample application :
Have fun.
Download Sample - 38KB Read Disclaimer Notice
- Set WindowStyle to Maximized : By this the Window will not show up any title bar. The window style will be transformed to a Box with border in it.
- Set WindowState to None : By this the window will be maximized to the whole screen and will show only the window and nothing else..
Oh.. Hold on ..Hold on... As I say, it hides the whole screen, it means it even will not show up the Taskbar or any external gadgets applied to your desktop if it is not set to Always on Top attribute to it.
Yes, this is the problem that I faced recently, when people wanted me to show the TaskBar even though the application should be Maximized.
Is it a Bug ?
If I am not wrong, WPF builds application based on the Screen Resolution. It produces DPI independent pixels. If you specify it to be full screen, it first gets the Resolution of the screen, and draws the pixel based on its own algorithm. Hence, when you specify it to Maximized, it takes up the whole screen, as otherwise some portion of the screen will be hidden outside the range of the boundary.
When you restore a WPF screen, it will also recalculate the work area based on the distance between the resolution boundaries and resize itself accordingly. No do you think Microsoft should really have an alternative state which show up the Taskbar as it does with normal windows ? I think yes.
The Solution
As I needed to do this, I have just tried out few workarounds to this myself.
Following Lester's Blog on this issue, I have to use
private static System.IntPtr WindowProc( System.IntPtr hwnd, int msg, System.IntPtr wParam, System.IntPtr lParam, ref bool handled) { switch (msg) { case 0x0024: WmGetMinMaxInfo(hwnd, lParam); handled = true; break; } return (System.IntPtr)0; } private static void WmGetMinMaxInfo(System.IntPtr hwnd, System.IntPtr lParam) { MINMAXINFO mmi = (MINMAXINFO)Marshal.PtrToStructure(lParam, typeof(MINMAXINFO)); // Adjust the maximized size and position to fit the work area of the correct monitor int MONITOR_DEFAULTTONEAREST =0x00000002; System.IntPtr monitor = MonitorFromWindow(hwnd, MONITOR_DEFAULTTONEAREST); if (monitor != System.IntPtr.Zero) { MONITORINFO monitorInfo = new MONITORINFO(); GetMonitorInfo(monitor, monitorInfo); RECT rcWorkArea = monitorInfo.rcWork; RECT rcMonitorArea = monitorInfo.rcMonitor; mmi.ptMaxPosition.x = Math.Abs(rcWorkArea.left - rcMonitorArea.left); mmi.ptMaxPosition.y = Math.Abs(rcWorkArea.top - rcMonitorArea.top); mmi.ptMaxSize.x = Math.Abs(rcWorkArea.right - rcWorkArea.left); mmi.ptMaxSize.y = Math.Abs(rcWorkArea.bottom - rcWorkArea.top); } Marshal.StructureToPtr(mmi, lParam, true); }
The call to API WmGetMinMaxInfo gets you the size of Maximize window for the current desktop.
[DllImport("user32")] internal static extern bool GetMonitorInfo(IntPtr hMonitor, MONITORINFO lpmi); [DllImport("User32")] internal static extern IntPtr MonitorFromWindow(IntPtr handle, int flags);
The call to GetMonitorInfo gets you a MONITORINFO object and if you see the code carefully it actually position the window in such a way that it absolutely resize itself to the height and width of the rectangular area.
To call this method, I can use SourceInitialized event which will eventually be called whenever the WindowState is modified.
void win_SourceInitialized(object sender, EventArgs e) { System.IntPtr handle = (new WinInterop.WindowInteropHelper(this)).Handle; WinInterop.HwndSource.FromHwnd(handle).AddHook(new WinInterop.HwndSourceHook(WindowProc)); }
Sounds good ?
Oh.. Lets give another easier solution that I have used. Its very simple.
Set properties for your window :
WindowStyle="None" WindowState="Maximized" ResizeMode="NoResize"
And go to your code and just resize your window based on PrimaryScreen width and height. Also make sure you do set the Left and Top of the window as well.
this.Width = System.Windows.SystemParameters.WorkArea.Width; this.Height = System.Windows.SystemParameters.WorkArea.Height; this.Left = 0; this.Top = 0; this.WindowState = WindowState.Normal;
Making WindowState.Normal will ensure that the default behaviour of the window is overridden and also makes the Taskbar Reappear.
I have included my sample application :
Have fun.
Download Sample - 38KB Read Disclaimer Notice
.NET Community Session : ASP.NET 4.0 In - Depth
Posted by
Abhishek Sur
Labels:
.NET 4.0,
ASP.NET 4.0,
Online Session
1 comments
I am going to speak on a Community Session Organized by DotNetFunda on ASP.NET 4.0 with Abhijit Jana.
ASP.NET 4.0 comes with lots of new features that enhance the developers to deal with ASP.NET applications easily and also core functionality to deal with both server side and client side of an ASP.NET application. These changes makes ASP.NET 4.0 applications more effective than the existing ASP.NET applications.
The Agenda for the Session :
Scheduled Date: 19-Sep-2010 (Sunday )
Scheduled Time: 2.00 PM to 4.30 PM
Live meeting URL: https://www.livemeeting.com/cc/mvp/
Meeting ID: Will be sent to your email id before the session scheduled date and time.
Is Paid?: No, It’s FREE Session
Is Registration required ? Yes . Please register here http://bit.ly/d6aVYM .
Disclaimer : http://www.abhisheksur.com/p/disclaimer.html Read Disclaimer Notice
ASP.NET 4.0 comes with lots of new features that enhance the developers to deal with ASP.NET applications easily and also core functionality to deal with both server side and client side of an ASP.NET application. These changes makes ASP.NET 4.0 applications more effective than the existing ASP.NET applications.
The Agenda for the Session :
![]() |
(Click to enlarge to Full Size) |
- What’s New in ASP.NET 4.0
- Improvement in Visual Studio 2010 for web development
- Empty Website project and Cleaner Web.Config
- MetaKeyword and MetaDescription enhancements
- Controlling ViewState mechanism
- Controlling ClientID generation for asp.net controls
- CompressionEnabled Session
- CSS Improvements and CSS Friendly Menu Control
- ListView rendering mechanism, Enhancement in Listview control
- Enhancement in RadioButtonList and CheckBoxList
- SEO Friendly URL (Web Form Routing)
- Permanent Redirection mechanism
- Improvements in Code Expressions
- Overview of Dynamic data
- Caching mechanism (Output Caching, Object caching etc.)
- ASP.NET Applications / Database deployment.
- Q & A
- Summary
Scheduled Date: 19-Sep-2010 (Sunday )
Scheduled Time: 2.00 PM to 4.30 PM
Live meeting URL: https://www.livemeeting.com/cc/mvp/
Meeting ID: Will be sent to your email id before the session scheduled date and time.
Is Paid?: No, It’s FREE Session
Is Registration required ? Yes . Please register here http://bit.ly/d6aVYM .
Disclaimer : http://www.abhisheksur.com/p/disclaimer.html Read Disclaimer Notice
Use of Expression Trees in .NET for Lambda Decomposition
Posted by
Abhishek Sur
on Monday, September 6, 2010
Labels:
.NET 3.5,
.NET 4.0,
C#,
CodeProject
4
comments
Expressions are the building block of any Lambda Expression. In C# 3.0, .NET framework has introduced a new technique to make use of Anonymous methods a better way. The "Little Gem" LINQ, uses Lambda expression extensively to invoke filter statements to IEnumerable objects and hence making the life for a programmer very easy. Simple lambda expressions like
may come very handy as we do not need to define a method extensively for such a small purpose. C# also introduced a couple of Generic delegates like Func<...>, Action<...> etc. in Base Class Library which lets you to pass any type of argument to a method body. The one with Func are for methods which require something to return while ones with Action dont need to return anything.
To learn more about LINQ please have a quick pick on my article on LINQ and Lambda Expressions.But if you have already read about the basics of LINQ, it is not all that is needed. Lambda expressions are cool and extensive use of Anonymous methods are truly awesome, but basically when I think of using Lambda Expression in my own application, I always think of extending the Lambda expressions a bit further so that I could use it dynamically during runtime and later on compile them to produce the actual anonymous delegates. In this article I will put forward the story of Linq and Lambda Expressions further and introduce you with Expression Trees and also show you to create Expression Trees dynamically during runtime.
What is an Expression Tree?
Simply speaking, an expression tree is nothing but the representation of a Lambda Expression in terms of .NET objects. The Expression is the main class that holds any expression in it and it lets us to divide the whole body of the Expression into smaller individual units. For instance :
In the above code the delegate Func can take the expression x=>x<5 which represents an anonymous method that takes an integer argument and returns if the value is less than 5.
Download Sample - 38 KB
Read Disclaimer Notice
x => x<5
may come very handy as we do not need to define a method extensively for such a small purpose. C# also introduced a couple of Generic delegates like Func<...>, Action<...> etc. in Base Class Library which lets you to pass any type of argument to a method body. The one with Func are for methods which require something to return while ones with Action dont need to return anything.
To learn more about LINQ please have a quick pick on my article on LINQ and Lambda Expressions.But if you have already read about the basics of LINQ, it is not all that is needed. Lambda expressions are cool and extensive use of Anonymous methods are truly awesome, but basically when I think of using Lambda Expression in my own application, I always think of extending the Lambda expressions a bit further so that I could use it dynamically during runtime and later on compile them to produce the actual anonymous delegates. In this article I will put forward the story of Linq and Lambda Expressions further and introduce you with Expression Trees and also show you to create Expression Trees dynamically during runtime.
What is an Expression Tree?
Simply speaking, an expression tree is nothing but the representation of a Lambda Expression in terms of .NET objects. The Expression
Func<int, bool> mydelegate = x => x < 5; Expression<Func<int, bool>> myexpressiondelegate = x => x < 5;
In the above code the delegate Func
Read Disclaimer Notice
Notion of System.Nullable - A Look
Posted by
Abhishek Sur
on Saturday, September 4, 2010
Labels:
.NET,
.NET 3.5,
.NET 4.0,
C#
2
comments
I love C# as a language. It has lots of flexibilities and hooks to turn around things that was impossible for other languages. One of such thing is the introduction of Nullable Types. In this post, I will discuss how you could use Nullable to make sure your code works well for null values in Value Types.
Why System.Nullable?
You must already know, System.Nullable is the only structure that supports the storage of Null as a valid range of values. Generally in case of structures it cannot store null values in it. Say for instance :
Int32 is a structure. When you specify
It will automatically assign 0 to x. This is same as
Generally structures always recursively call default to initialize the value of it. Say for instance :
I have defined a custom structure MyCustomStructure. If you declare an object of it like :
It will assign myobj to its default. You cannot assign null to myobj. The only way out to this situation is Nullable.
Hence you can see, you should always use Nullable for a structure if you want to store the value of null to a structure. For data centric applications, we generally need to keep a variable out of the scope of valid values so that the variable can have a value which might identify the value as nothing. Null is taken as nothing, but in case of normal structures it does not allow us to do that. If you store nothing to an integer, it will automatically initialize to 0. But for any application 0 might be a valid value. So you cannot exclude the 0 from the range of data. Hence you need Nullable to workaround this issue.
Read Disclaimer Notice
Why System.Nullable?
You must already know, System.Nullable is the only structure that supports the storage of Null as a valid range of values. Generally in case of structures it cannot store null values in it. Say for instance :
Int32 is a structure. When you specify
int x;
It will automatically assign 0 to x. This is same as
int x = default(int);
Generally structures always recursively call default to initialize the value of it. Say for instance :
struct MyCustomStructure { public int m; public int n; }
I have defined a custom structure MyCustomStructure. If you declare an object of it like :
MyCustomStructure myobj;
It will assign myobj to its default. You cannot assign null to myobj. The only way out to this situation is Nullable.
MyCustomStructure myobj = null; //throws exception Nullable<MyCustomStructure> myobj = null; //works fine
Hence you can see, you should always use Nullable for a structure if you want to store the value of null to a structure. For data centric applications, we generally need to keep a variable out of the scope of valid values so that the variable can have a value which might identify the value as nothing. Null is taken as nothing, but in case of normal structures it does not allow us to do that. If you store nothing to an integer, it will automatically initialize to 0. But for any application 0 might be a valid value. So you cannot exclude the 0 from the range of data. Hence you need Nullable to workaround this issue.
Read Disclaimer Notice
Implementation of an Observer
Posted by
Abhishek Sur
on Sunday, August 29, 2010
Hi Folks,
As few people told me to implement an observer in my last post where I just showed how to use it, here is the post where I am going to clear out confusions for you. If you have read my other post, you might already know what is an Observer and why it is required. Lets recap this a bit more.
An observer is a container which observes each element individually and notifies you when the object state is modified. The observer should contain methods that enables you to subscribe or unsubscribe individually so that when you subscribe for a notification, it will keep on creating notification until you explicitly unsubscribe the Observer.
In .NET base class library, there are two interfaces introduced viz, IObservable and IObserver. These interfaces gives you a standard to develop Observable pattern and also recommends you to use it rather than doing it of your own. Microsoft also builds forth its Reactive Framework (I will discuss about it later) based on Observer pattern and lets us use it when Observer is required.
In this post, I will discuss how you could use IObserver and IObservable to implement you own notifiers.
Download Sample - 33KB
Read Disclaimer Notice
As few people told me to implement an observer in my last post where I just showed how to use it, here is the post where I am going to clear out confusions for you. If you have read my other post, you might already know what is an Observer and why it is required. Lets recap this a bit more.
An observer is a container which observes each element individually and notifies you when the object state is modified. The observer should contain methods that enables you to subscribe or unsubscribe individually so that when you subscribe for a notification, it will keep on creating notification until you explicitly unsubscribe the Observer.
In .NET base class library, there are two interfaces introduced viz, IObservable
In this post, I will discuss how you could use IObserver and IObservable to implement you own notifiers.
Download Sample - 33KB
Read Disclaimer Notice
Working with CollectionView in WPF(Filter,Sort, Group, Navigate)
Posted by
Abhishek Sur
on Wednesday, August 25, 2010
Labels:
.NET 3.5,
.NET 4.0,
C#,
CodeProject,
WPF
2
comments
If you are working with WPF for long, you might already have come across with ICollectionView. It is the primary Data object for any WPF list controls (like ComboBox, ListBox, ListView etc) that allows flexibilities like Sorting, Filtering, Grouping, Current Record Management etc. Thus it ensures that all the related information like filtering, sorting etc is decoupled from the actual control. It is been very popular to those working with data object because of inbuilt support for all WPF List controls. So I thought I would consider to describe it a bit so that people might easily plug in the same to their own solution.
What is a CollectionView ?
It is a layer that runs over the Data Objects which allows you to define rules for Sorting, Filtering, Grouping etc and manipulate the display of data rather than modifying the actual data objects. Therefore in other words, a CollectionView is a class which takes care of the View totally and giving us the capability to handle certain features incorporated within it.
How to Get a CollectionView ?
Practically speaking, getting a CollectionView from an Enumerable the most easiest thing I ever seen in WPF. Just you need to pass the Enumerable to CollectionViewSource.GetDefaultView. Thus rather than defining
you need to write :
The List will get the CollectionView it requires.
So the CollectionView actually separates the View object List Control with the actual DataSource and hence gives an interface to manipulate the data before reflecting to the View objects. Now let us look how to implement the basic features for the ICollectionView.
Download Sample - 74KB
Read Disclaimer Notice
What is a CollectionView ?
It is a layer that runs over the Data Objects which allows you to define rules for Sorting, Filtering, Grouping etc and manipulate the display of data rather than modifying the actual data objects. Therefore in other words, a CollectionView is a class which takes care of the View totally and giving us the capability to handle certain features incorporated within it.
How to Get a CollectionView ?
Practically speaking, getting a CollectionView from an Enumerable the most easiest thing I ever seen in WPF. Just you need to pass the Enumerable to CollectionViewSource.GetDefaultView. Thus rather than defining
this.ListboxControl.ItemsSource = this.Source;
you need to write :
this.ListboxControl.ItemsSource = CollectionViewSource.GetDefaultView(this.Source);
The List will get the CollectionView it requires.
So the CollectionView actually separates the View object List Control with the actual DataSource and hence gives an interface to manipulate the data before reflecting to the View objects. Now let us look how to implement the basic features for the ICollectionView.
Download Sample - 74KB
Read Disclaimer Notice
Author's new book
Abhishek authored one of the best selling book of .NET. It covers ASP.NET, WPF, Windows 8, Threading, Memory Management, Internals, Visual Studio, HTML5, JQuery and many more...
Grab it now !!!
Grab it now !!!